Before we dive into fixing the issue of “converting CSV to string causing memory issues,” I recommend trying my online CSV-to-string converter. It can help you convert CSV files to strings, but please note that it might slow down or cause your browser to lag. This is not the ultimate solution but might be helpful for quick tasks.
Uploading CSV file…
Processing CSV file…
Now, let’s explore the reasons behind this issue and how to resolve it.
Why Converting CSV to String Causes Memory Issues in Java?
When converting a CSV file to a string in Java, you might encounter memory issues due to several factors:
- Large File Size: If the CSV file is substantial, loading the entire file into a single string can consume a significant amount of memory. Java's memory allocation for strings is limited by the JVM's heap size. Trying to load a massive file into memory can lead to an OutOfMemoryError.
- Inefficient Memory Usage: Concatenating strings in Java using the + operator or String.concat() can be inefficient. Each concatenation operation creates a new string object, leading to excessive memory usage and slow performance. Using StringBuilder or StringBuffer for concatenation is a more memory-efficient approach.
- Memory Fragmentation: When dealing with very large strings, the JVM might struggle with memory fragmentation. Free memory might get divided into small blocks, making it challenging to allocate contiguous space for a large string.
- Improper Stream Handling: If you're reading the CSV file using streams, ensure that you close them properly. Failing to close streams can lead to memory leaks and other resource management issues.
How to Fix the Issue Using Visual Studio Code (VS Code) and Java
Here’s a step-by-step guide to fixing the 'Converting CSV to String Causing Memory Issues' problem using VS Code as an example:
- Install the Java Extension Pack
- Open VS Code.
- Go to the Extensions view by clicking the Extensions icon in the Activity Bar on the side or by pressing Ctrl+Shift+X.
- Search for "Extension Pack for Java" and install it. This pack includes the essential extensions for Java development.
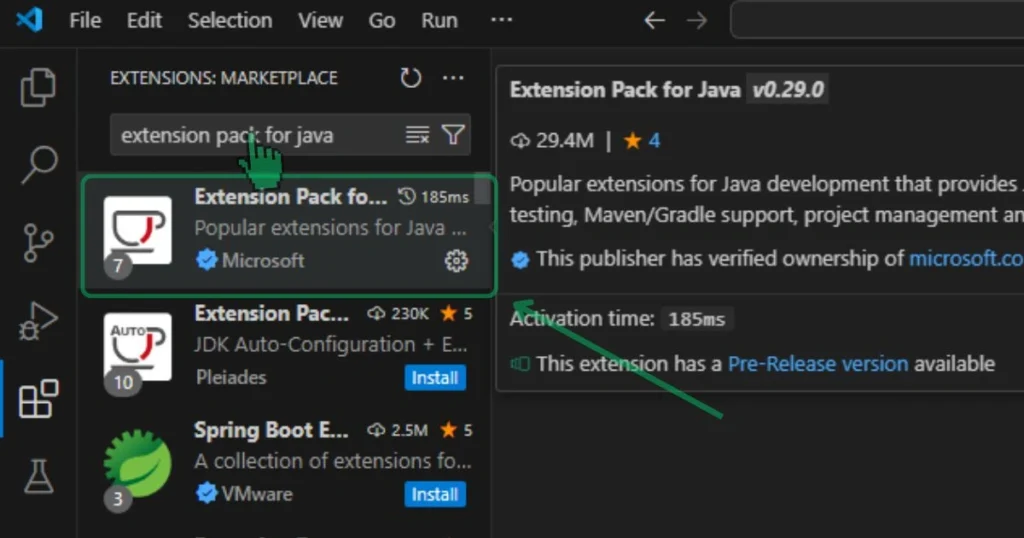
- Create a New Java Project
- Open the Command Palette by pressing Ctrl+Shift+P.
- Type and select "Java: Create Java Project."
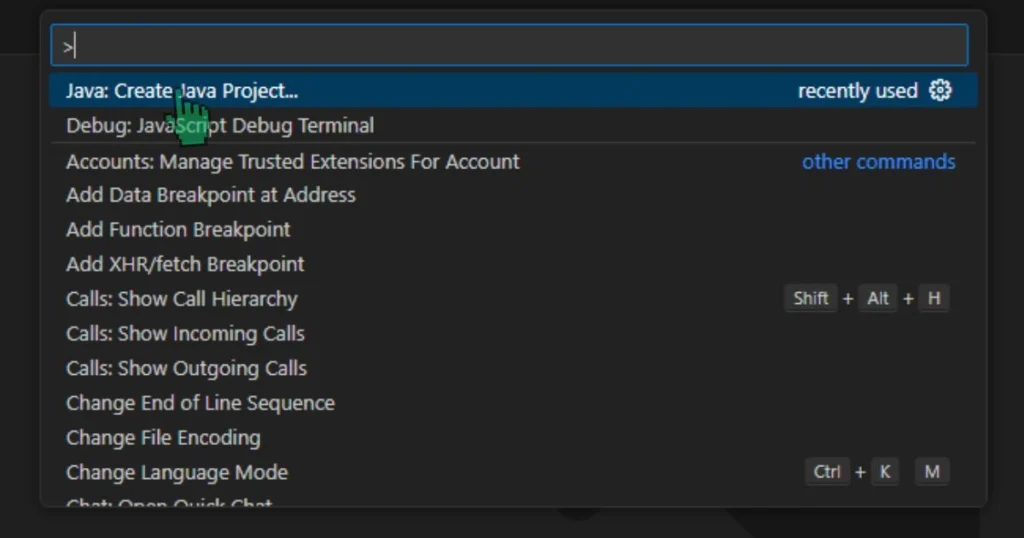
- Choose “No Build Tools.”
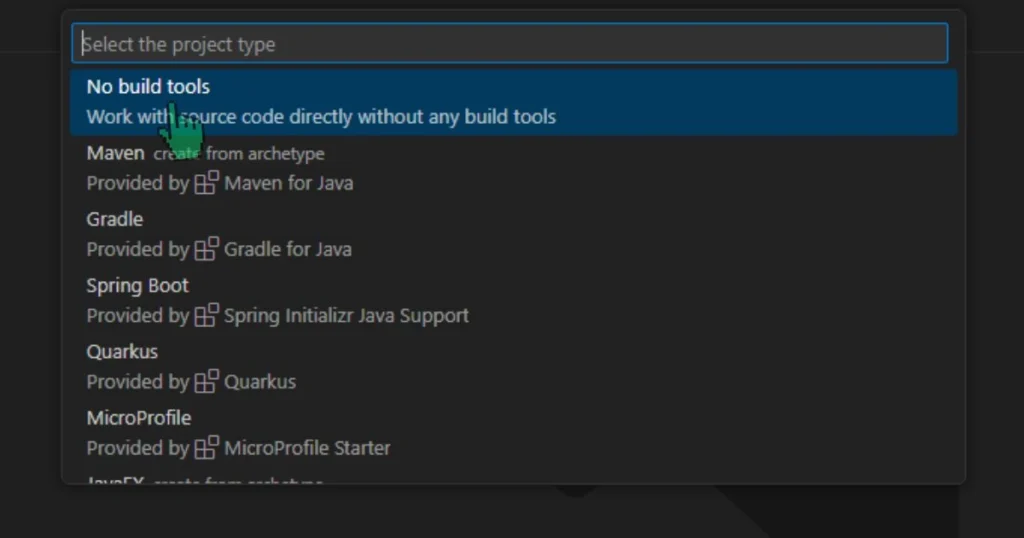
- Specify the location to save your project.
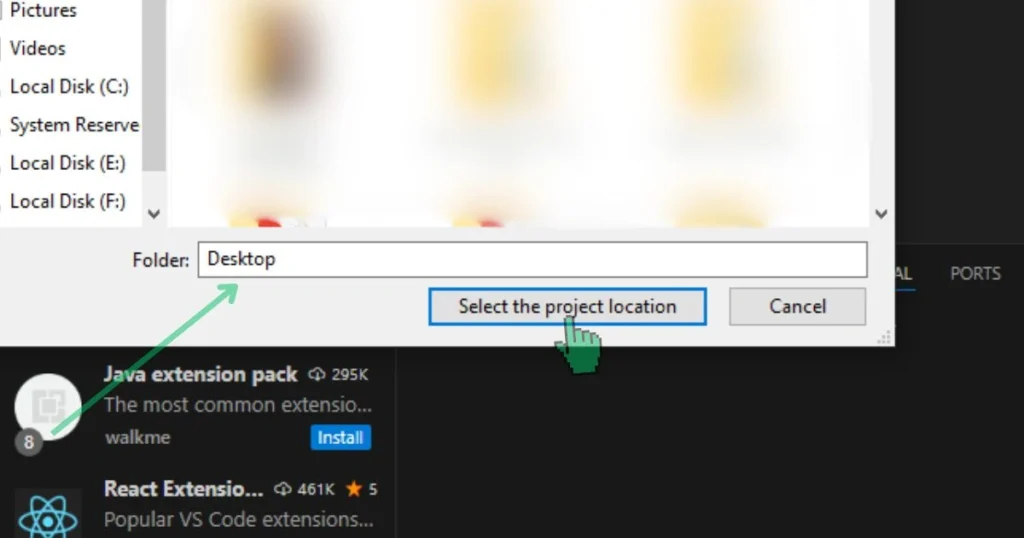
- Enter a name for your project and press Enter.
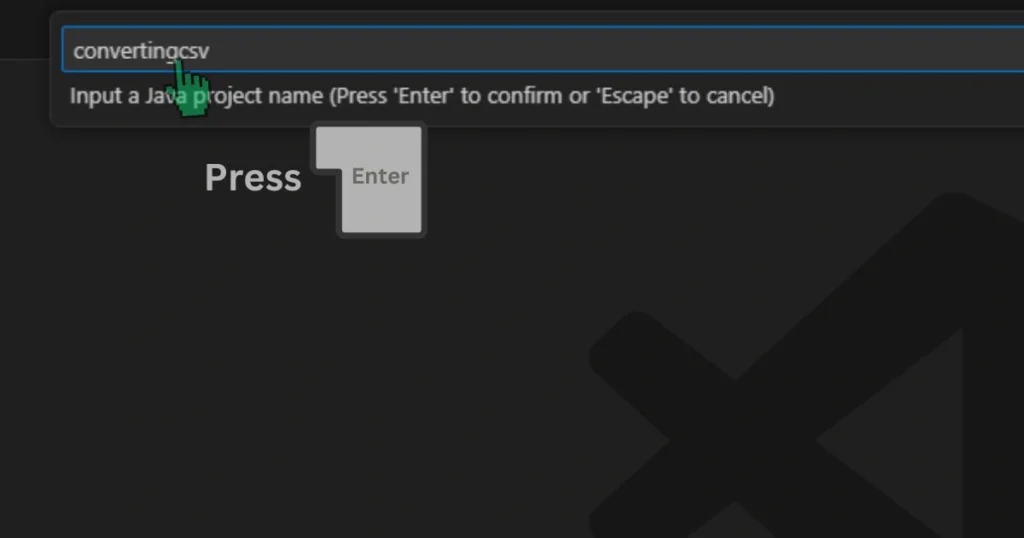
- Use BufferedReader and BufferedWriter for Efficient Memory Usage
- Navigate to src > App.java and replace the existing code with the following:
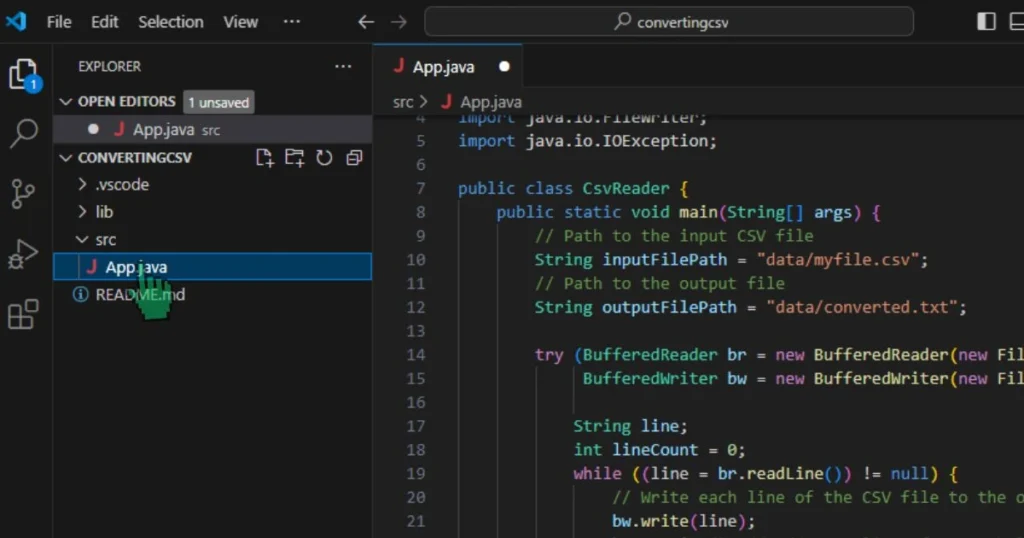
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class CsvReader {
public static void main(String[] args) {
// Path to the input CSV file
String inputFilePath = "data/myfile.csv";
// Path to the output file
String outputFilePath = "data/converted.txt";
try (BufferedReader br = new BufferedReader(new FileReader(inputFilePath));
BufferedWriter bw = new BufferedWriter(new FileWriter(outputFilePath))) {
String line;
int lineCount = 0;
while ((line = br.readLine()) != null) {
// Write each line of the CSV file to the output file
bw.write(line);
bw.newLine(); // Add a newline after each line
// Debugging: Print the number of lines processed
lineCount++;
if (lineCount % 100 == 0) {
System.out.println("Processed " + lineCount + " lines...");
}
}
System.out.println("Data successfully written to " + outputFilePath);
System.out.println("Total lines processed: " + lineCount);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Click CNTR+S to save the code.
This code efficiently handles the conversion of CSV lines to a text file. It uses BufferedReader and BufferedWriter for better performance and memory management. The program also includes debugging statements to track the progress of line processing.
- Prepare Your Files
Create a new folder in your project and name it data. Go to that folder and paste your CSV file there.
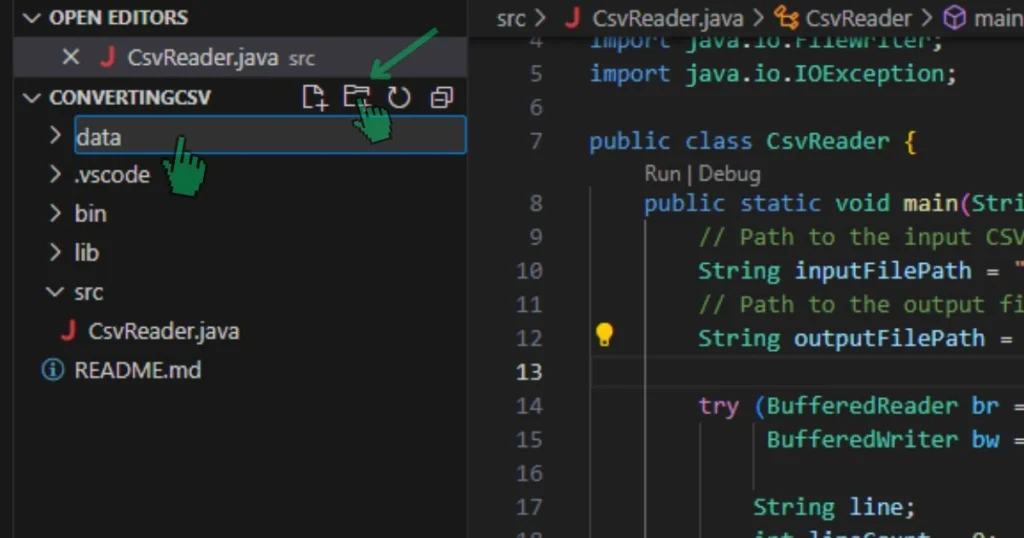
Type the CSV file name in "data/mydata.csv" and specify a name for the output file in "data/converted.txt". Your text file will be found in the data folder that you created.
Ensure there are no trailing slashes after the file format (e.g., converted.txt/), and use double quotes (").
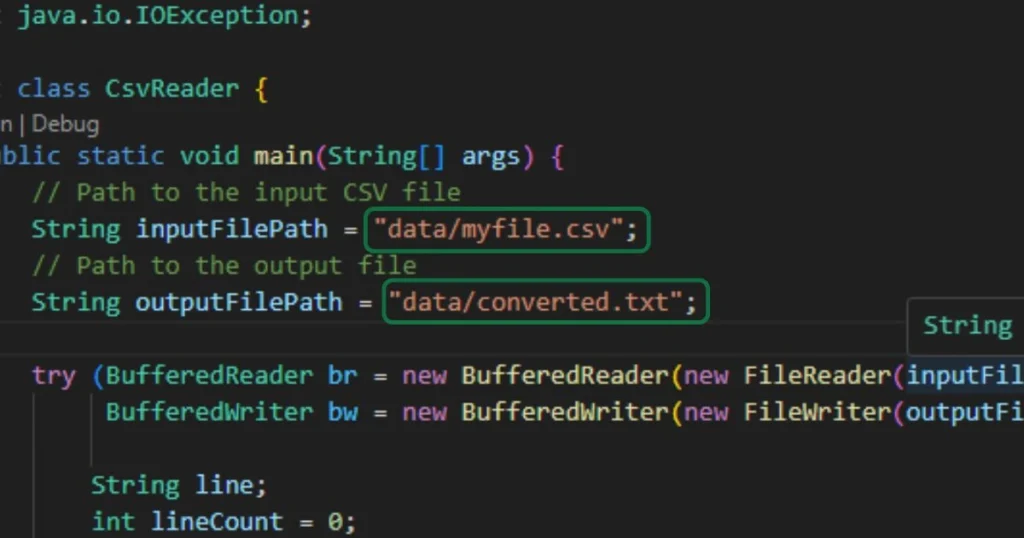
- Run the Program
Click RUN to start converting your CSV file. You will find a .txt file at the location you specified, you can use CommaTool.com to convert a CSV file into column if your data has a single column.
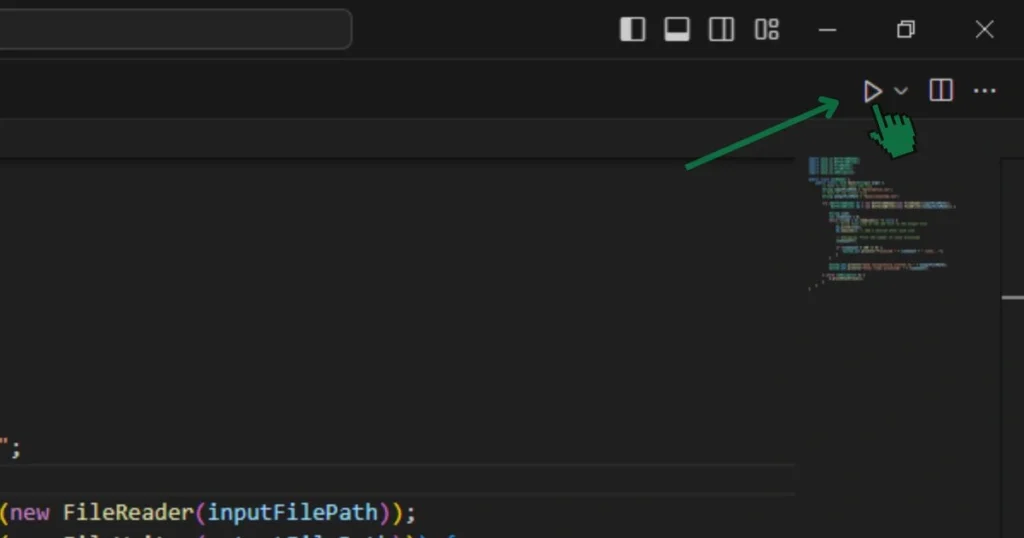
In this example, a CSV file containing 3,000,000 rows and 7 columns was converted in less than 2 minute.
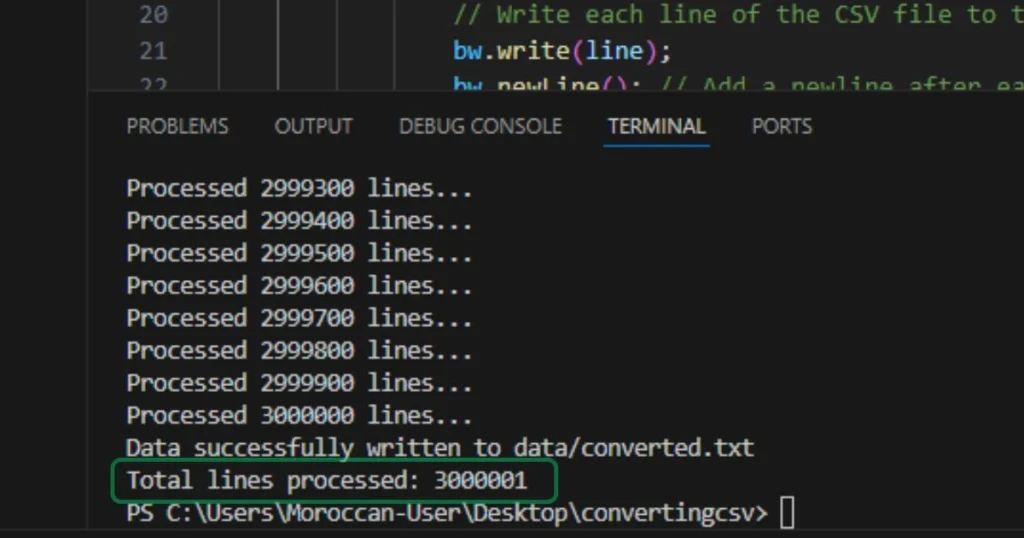
Conclusion
Handling large CSV files in Java can be challenging due to memory limitations and inefficiencies in string handling. By using efficient methods such as BufferedReader and BufferedWriter, you can mitigate memory issues and achieve better performance. This approach helps manage memory usage more effectively and avoids potential pitfalls like OutOfMemoryError and memory fragmentation.
Remember, while tools like the online CSV-to-string converter can be useful for quick tasks, implementing a robust solution within your Java code is crucial for handling large datasets efficiently. By following the steps outlined in this guide, you can successfully convert large CSV files into text files without running into significant memory issues.
If you encounter any further challenges or have additional questions, feel free to reach out or leave a comment below.